Mastering React Router has become essential for seamless page switching and routing in React applications. Introduced in 2014, React Router has grown in tandem with React, becoming a cornerstone of the React ecosystem. This guide offers a comprehensive exploration of React Router, covering fundamental principles and advanced implementation strategies.
We’ll begin by exploring the fundamental principles of declarative routing in React and why React Router stands out among other routing libraries. Then, we’ll dive into creating a basic application that demonstrates various routing features. Finally, we’ll focus on developing an elegant, secure, and reusable routing component that aligns seamlessly with React’s coding guidelines and style.
By the end of this tutorial, you’ll have a solid understanding of how to implement efficient and maintainable routing solutions in your React applications. Let’s embark on this journey to master React Router and elevate your development skills!
Declarative Routing: The Foundation of React Router
Declarative routing is the cornerstone of React Router’s design philosophy. This approach aligns perfectly with React’s component-based architecture, allowing developers to define routes as components within their application structure. The beauty of this method lies in its simplicity and consistency with React’s overall coding paradigm.
In declarative routing, routes are essentially React components that associate specific web addresses with particular pages or components. This integration leverages React’s powerful rendering engine and conditional logic, enabling developers to activate or deactivate routes programmatically based on various conditions.
The advantages of declarative routing in React Router include:
- Intuitive Structure: Routes are defined using JSX syntax, making them easy to read and understand.
- Seamless Integration: Routes behave like any other React component, allowing for consistent state management and prop passing.
- Dynamic Routing: Conditional logic can be applied to routes, enabling dynamic routing based on application state or user permissions.
- Code Organization: Routes can be organized and nested logically, improving code maintainability.
React Router v6, the latest major version, has introduced several enhancements to the declarative routing model. Key changes include:
- The replacement of the
<Switch>
component with<Routes>
. - Introduction of the
useRoutes()
hook for defining routes as plain objects. - A stricter requirement that all children of
<Routes>
must be<Route>
components.
These changes have further streamlined the routing process and improved the overall developer experience. As we progress through this tutorial, we’ll explore how to leverage these new features to create robust and efficient routing solutions.
Setting Up a Basic React Application with Routing
To demonstrate the power and flexibility of React Router, we’ll create a simple React application that showcases various routing features. We’ll use Vite as our build tool for its speed and simplicity. Follow these steps to set up your project:
- Open your terminal and run the following command to create a new React project with TypeScript support:
npm create vite@latest redirect-app -- --template react-ts
- Navigate to the project directory:
cd redirect-app
- Install the necessary dependencies:
npm install react-router-dom@6
- Start the development server:
npm run dev
With our basic React application set up, we can now start implementing routing. Let’s begin by creating a simple navigation structure and defining some routes.
First, let’s create a basic layout component that includes navigation:
// src/components/Layout.tsx
import { Link, Outlet } from 'react-router-dom';
export function Layout() {
return (
<div>
<nav>
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/about">About</Link></li>
<li><Link to="/dashboard">Dashboard</Link></li>
</ul>
</nav>
<Outlet />
</div>
);
}
Now, let’s define our routes in the main App component:
// src/App.tsx
import { BrowserRouter as Router, Routes, Route } from 'react-router-dom';
import { Layout } from './components/Layout';
import { Home } from './pages/Home';
import { About } from './pages/About';
import { Dashboard } from './pages/Dashboard';
function App() {
return (
<Router>
<Routes>
<Route path="/" element={<Layout />}>
<Route index element={<Home />} />
<Route path="about" element={<About />} />
<Route path="dashboard" element={<Dashboard />} />
</Route>
</Routes>
</Router>
);
}
export default App;
This basic setup demonstrates the declarative nature of React Router. We’ve defined our routes as components, nested them logically, and used the <Outlet />
component to render child routes within our layout.
Implementing Protected Routes
One of the most common requirements in web applications is implementing protected routes – pages that should only be accessible to authenticated users. React Router doesn’t provide this functionality out of the box, but we can easily create a custom component to handle this logic.
Let’s create a ProtectedRoute
component that checks if a user is authenticated before rendering a route:
// src/components/ProtectedRoute.tsx
import { ReactNode } from 'react';
import { Navigate } from 'react-router-dom';
interface ProtectedRouteProps {
isAuthenticated: boolean;
children: ReactNode;
}
export function ProtectedRoute({ isAuthenticated, children }: ProtectedRouteProps) {
if (!isAuthenticated) {
return <Navigate to="/login" replace />;
}
return <>{children}</>;
}
Now we can use this component to wrap our protected routes:
// src/App.tsx
import { BrowserRouter as Router, Routes, Route } from 'react-router-dom';
import { Layout } from './components/Layout';
import { Home } from './pages/Home';
import { About } from './pages/About';
import { Dashboard } from './pages/Dashboard';
import { Login } from './pages/Login';
import { ProtectedRoute } from './components/ProtectedRoute';
function App() {
const isAuthenticated = /* Your authentication logic here */;
return (
<Router>
<Routes>
<Route path="/" element={<Layout />}>
<Route index element={<Home />} />
<Route path="about" element={<About />} />
<Route path="login" element={<Login />} />
<Route
path="dashboard"
element={
<ProtectedRoute isAuthenticated={isAuthenticated}>
<Dashboard />
</ProtectedRoute>
}
/>
</Route>
</Routes>
</Router>
);
}
export default App;
This implementation ensures that users can only access the Dashboard if they’re authenticated. If not, they’ll be redirected to the login page.
Advanced Routing Techniques
As your application grows in complexity, you might need more advanced routing techniques. React Router v6 provides several powerful features to handle complex routing scenarios. Let’s explore some of these techniques:
1. Nested Routes
Nested routes allow you to create more complex UI hierarchies. For example, you might have a user profile page with sub-sections:
<Route path="users/:id" element={<UserProfile />}>
<Route path="posts" element={<UserPosts />} />
<Route path="followers" element={<UserFollowers />} />
</Route>
2. Dynamic Routes
Dynamic routes allow you to create paths with parameters. You can access these parameters using the useParams
hook:
import { useParams } from 'react-router-dom';
function UserProfile() {
const { id } = useParams();
// Use the id to fetch user data
}
3. Programmatic Navigation
Sometimes you need to navigate programmatically, such as after form submission. Use the useNavigate
hook for this:
import { useNavigate } from 'react-router-dom';
function LoginForm() {
const navigate = useNavigate();
const handleSubmit = (event) => {
event.preventDefault();
// Perform login logic
navigate('/dashboard');
};
// Form JSX
}
4. Route Config Objects
For larger applications, you might prefer to define your routes as JavaScript objects. React Router v6 provides the useRoutes
hook for this purpose:
import { useRoutes } from 'react-router-dom';
const routes = [
{
path: '/',
element: <Layout />,
children: [
{ index: true, element: <Home /> },
{ path: 'about', element: <About /> },
{ path: 'dashboard', element: <Dashboard /> },
],
},
];
function App() {
const element = useRoutes(routes);
return element;
}
These advanced techniques provide the flexibility and power needed to handle complex routing scenarios in large-scale React applications.
Optimizing Performance with React Router
While React Router provides powerful routing capabilities, it’s important to optimize its usage to ensure your application performs well, especially as it grows in size and complexity. Here are some strategies to enhance performance when using React Router:
1. Code Splitting
Code splitting is a technique that allows you to split your application into smaller chunks and load them on demand. This can significantly improve initial load times. React’s lazy
and Suspense
can be used in conjunction with React Router for effective code splitting:
import React, { lazy, Suspense } from 'react';
import { BrowserRouter as Router, Routes, Route } from 'react-router-dom';
const Home = lazy(() => import('./pages/Home'));
const About = lazy(() => import('./pages/About'));
const Dashboard = lazy(() => import('./pages/Dashboard'));
function App() {
return (
<Router>
<Suspense fallback={<div>Loading...</div>}>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/about" element={<About />} />
<Route path="/dashboard" element={<Dashboard />} />
</Routes>
</Suspense>
</Router>
);
}
2. Memoization
Use React’s useMemo
and useCallback
hooks to memoize expensive computations or callback functions that are passed as props to route components. This can prevent unnecessary re-renders:
import React, { useMemo } from 'react';
import { useParams } from 'react-router-dom';
function UserProfile() {
const { id } = useParams();
const userData = useMemo(() => fetchUserData(id), [id]);
return <div>{/* Render user profile */}</div>;
}
3. Avoid Unnecessary Re-renders
Be cautious when using hooks like useLocation
or useParams
in parent components. These hooks can cause re-renders on every navigation. Instead, use them in the specific components that need the route information.
4. Preloading Routes
For critical routes, you can implement preloading to fetch the route component before the user navigates to it. This can be done on hover or when the user shows intent to navigate:
import { Link, useNavigate } from 'react-router-dom';
function NavLink({ to, children }) {
const navigate = useNavigate();
const handleMouseEnter = () => {
// Preload the route component
import(`./pages${to}`).then(() => {
console.log(`Preloaded: ${to}`);
});
};
return (
<Link to={to} onMouseEnter={handleMouseEnter}>
{children}
</Link>
);
}
By implementing these optimization techniques, you can ensure that your React application with React Router remains performant and provides a smooth user experience, even as it scales.
Mastering React Router for Efficient Web Development
Throughout this comprehensive guide, we’ve explored the intricacies of React Router, from its fundamental concepts to advanced implementation techniques. We’ve learned how to set up basic routing, implement protected routes, handle complex routing scenarios, and optimize performance.
Key takeaways from this tutorial include:
- Understanding the declarative nature of React Router and its alignment with React’s component-based architecture.
- Implementing basic routing structures and nested routes for complex UI hierarchies.
- Creating protected routes to secure parts of your application.
- Utilizing advanced features like dynamic routes, programmatic navigation, and route config objects.
- Optimizing performance through code splitting, memoization, and preloading techniques.
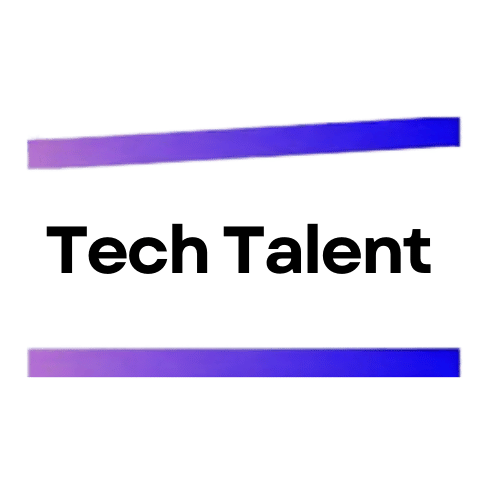
Explore TechTalent: Elevate Your Tech Career
Ready to take your interactive walkthrough skills to the next level?
TechTalent offers opportunities to certify your skills, connect with global tech professionals, and explore interactive design and development.
Join today and be part of shaping the future of interactive walkthroughs!