JavaScript arrays are powerful data structures that offer a plethora of built-in methods to manipulate and interact with data. In this comprehensive guide, we’ll delve into the intricacies of both destructive and non-destructive array methods, exploring their functionalities and practical applications. By the end of this article, you’ll have a solid grasp of these essential tools in your JavaScript arsenal, enabling you to write more efficient and elegant code.
Destructive Array Methods: Reshaping Your Data
Destructive array methods are the heavy hitters of JavaScript array manipulation. These methods alter the original array, providing a direct and often more memory-efficient way to modify your data. Let’s explore some of the most commonly used destructive methods:
- pop(): This method removes the last element from an array and returns it. It’s like plucking the cherry off the top of your sundae – the sundae (array) is forever changed.
- push(): The antithesis of pop(), push() adds one or more elements to the end of an array. It’s akin to adding toppings to your ice cream – each new element finds its place at the end.
- shift(): This method removes the first element from an array and returns it. Think of it as removing the bottom card from a deck – the whole stack shifts down.
- unshift(): The counterpart to shift(), unshift() adds one or more elements to the beginning of an array. It’s like dealing new cards to the top of the deck.
- splice(): The Swiss Army knife of array methods, splice() can add or remove elements from any position in the array. It’s incredibly versatile, allowing you to perform a complex array of surgeries with ease.
These destructive methods are invaluable when you need to modify your data in place, saving memory and potentially improving performance in certain scenarios. However, it’s crucial to use them judiciously, as they can lead to unexpected side effects if not handled carefully.
Non-Destructive Array Methods: Preserving the Original
Non-destructive array methods offer a safer alternative when you want to manipulate array data without altering the original array. These methods return a new array or value, leaving the source untouched. Let’s examine some key non-destructive methods:
- concat(): This method merges two or more arrays, creating a new array without modifying the originals. It’s like creating a playlist by combining songs from different albums.
- slice(): Slice() extracts a portion of an array, returning a new array containing the selected elements. It’s akin to cutting a slice of cake – you get a piece, but the cake remains intact.
- fill(): This method fills all the elements of an array with a static value. While it modifies the array in place, it’s often used to create new arrays with predetermined values.
Non-destructive methods are particularly useful when working with immutable data patterns or when you need to preserve the original array for future use. They provide a safe way to create new data structures based on existing arrays without risking unintended modifications.
Joining Array Elements: From Array to String
Sometimes, you need to convert an array into a string representation. The join() method serves this purpose perfectly:
- join(): This method concatenates all elements of an array into a string, with an optional separator. It’s like stringing beads together to form a necklace – each bead (element) is connected, forming a cohesive whole.
The join() method is incredibly useful for creating comma-separated lists, constructing URLs, or any scenario where you need to represent array data as a single string. Its non-destructive nature ensures that your original array remains intact, making it a safe choice for various applications.
Iterating and Processing Array Elements
JavaScript provides a rich set of methods for iterating over arrays and processing their elements. These methods offer more expressive and often more efficient alternatives to traditional loops.
Let’s explore some of the most powerful iteration methods:
- includes(): This method checks if an array contains a specific element, returning a boolean. It’s like searching for a specific book in a library – you either find it or you don’t.
- every(): Tests whether all elements in the array pass a provided test. It’s akin to a quality control check where every item must meet a certain standard.
- some(): Similar to every(), but returns true if at least one element passes the test. It’s like finding a needle in a haystack – you only need one match.
- find(): Returns the first element in the array that satisfies a provided testing function. It’s like a treasure hunt where you stop at the first gem you find.
- findIndex(): Similar to find(), but returns the index of the first matching element instead of the element itself.
- indexOf(): Returns the first index at which a given element can be found in the array. It’s like finding the page number of a specific topic in a book’s index.
- forEach(): Executes a provided function once for each array element. It’s like giving instructions to each person in a line – everyone gets their turn.
- map(): Creates a new array with the results of calling a provided function on every element in the array. It’s like a magical machine that transforms each item that passes through it.
- reduce(): Executes a reducer function on each element of the array, resulting in a single output value. It’s like distilling a complex recipe down to its essence.
- flat(): Creates a new array with all sub-array elements concatenated into it recursively up to the specified depth. It’s like unfolding a complex origami structure into a flat sheet.
- flatMap(): A combination of map() followed by flat() of depth 1. It’s like sorting and flattening a pile of nested boxes in one go.
These iteration methods provide powerful tools for processing array data, enabling you to write more declarative and readable code. By leveraging these methods, you can often avoid explicit loops and create more maintainable and efficient JavaScript programs.
Harnessing the Power of JavaScript Array Methods
JavaScript array methods offer a rich toolkit for manipulating and processing data. From destructive methods that modify arrays in place to non-destructive methods that create new data structures, and from simple joining operations to complex iterations, these methods provide elegant solutions to a wide range of programming challenges.
By mastering these array methods, you’ll be able to write more expressive, efficient, and maintainable code. Remember to choose the appropriate method based on your specific needs – whether you need to modify data in place, create new structures, or process elements without side effects.
As you continue your journey in JavaScript development, keep exploring and experimenting with these array methods. They are fundamental tools that will serve you well in countless scenarios, from simple data manipulation tasks to complex algorithmic challenges.
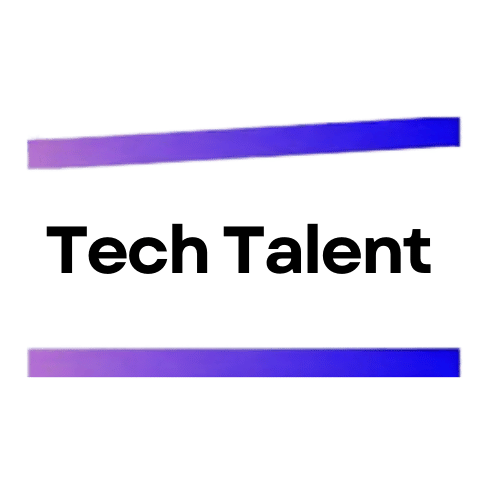
Explore TechTalent: Elevate Your Tech Career
Ready to take your interactive walkthrough skills to the next level?
TechTalent offers opportunities to certify your skills, connect with global tech professionals, and explore interactive design and development.
Join today and be part of shaping the future of interactive walkthroughs!