In the ever-evolving landscape of software engineering, C++ and C# stand out as two prominent programming languages with a rich history and widespread adoption. To truly understand their core differences, we must first delve into their origins and evolution.
C++: The Pioneer of Object-Oriented Programming
C++ emerged in the early 1980s as the brainchild of Danish computer scientist Bjarne Stroustrup. His vision was to create a language that combined the efficiency of C with the object-oriented paradigm of Simula. The result was “C with Classes,” which later evolved into C++.
Key milestones in C++ development:
- 1979: Stroustrup begins to work on “C with Classes”
- 1983: The name changes to C++
- 1985: First commercial release of C++
- 1998: First ISO standardization (C++98)
- 2011: Major update with C++11, introducing lambda expressions and smart pointers
- 2020: C++20 release, adding concepts and modules
C#: Microsoft’s Answer to Java
C# (pronounced “C sharp”) was developed by Microsoft in the early 2000s as part of its .NET framework. Led by Anders Hejlsberg, the team aimed to create a modern, object-oriented language that would compete with Java while addressing some of its perceived shortcomings.
Significant events in C# history:
- 2002: Initial release of C# 1.0
- 2005: C# 2.0 introduces generics
- 2007: C# 3.0 adds LINQ (Language Integrated Query)
- 2010: C# 4.0 introduces dynamic binding
- 2020: C# 9.0 brings record types and pattern-matching enhancements
Understanding this historical context is crucial as it illuminates the design philosophies and trade-offs made in each language’s development, which continue to influence their strengths and weaknesses today.
Core Language Features and Paradigms
While both C++ and C# share a common ancestor in C and support object-oriented programming, they differ significantly in their approach to language features and programming paradigms. These differences are at the core of what sets these languages apart and influence their suitability for various types of projects.
C++: Multi-paradigm Flexibility
C++ is renowned for its multi-paradigm approach, supporting:
- Procedural programming
- Object-oriented programming
- Generic programming
- Functional programming (to some extent)
This flexibility allows developers to choose the most appropriate paradigm for each part of their application. However, it also increases complexity and the potential for writing convoluted code.
Key C++ features:
- Multiple inheritance
- Templates for generic programming
- Operator overloading
- Low-level memory manipulation
- RAII (Resource Acquisition Is Initialization) for resource management
C#: Managed Code and .NET Integration
C# was designed with a focus on productivity and safety, emphasizing:
- Strong type safety
- Garbage collection
- Seamless integration with the .NET framework
While primarily object-oriented, C# has evolved to support functional programming concepts and asynchronous programming patterns.
Distinctive C# features:
- Properties and events
- LINQ for data querying
- Async/await for asynchronous programming
- Reflection for runtime type inspection
- Extension methods
These core language features and paradigms significantly influence the development experience and the types of applications for which each language is best suited. Understanding these fundamental differences is crucial for making informed decisions about which language to use for a given project.
Memory Management and Performance
One of the most significant distinctions between C++ and C# lies in their approaches to memory management and the resulting impact on performance. This aspect often plays a crucial role in determining which language is more suitable for a particular project, especially when dealing with resource-intensive applications or systems with limited hardware capabilities.
C++: Manual Memory Management and Raw Performance
C++ provides developers with direct control over memory allocation and deallocation, which can lead to superior performance when managed correctly. However, this power comes with increased responsibility and potential pitfalls:
- Manual memory management: Developers must explicitly allocate and free memory using
new
anddelete
operators. - RAII (Resource Acquisition Is Initialization): A technique used to manage resources automatically through object lifetimes.
- Smart pointers: Modern C++ provides smart pointers (
unique_ptr
,shared_ptr
,weak_ptr
) to help automate memory management. - Stack vs. Heap allocation: C++ allows fine-grained control over where objects are allocated, enabling performance optimizations.
The manual approach to memory management in C++ can lead to issues such as memory leaks, dangling pointers, and buffer overflows if not handled carefully. However, it also allows for highly optimized code that can achieve maximum performance.
C#: Managed Memory and Garbage Collection
C# takes a different approach, utilizing a managed memory model with automatic garbage collection:
- Garbage Collection (GC): Automatically frees memory that is no longer in use, reducing the risk of memory leaks.
- Value types vs. Reference types: C# distinguishes between stack-allocated value types and heap-allocated reference types.
- Disposable pattern: Used for managing unmanaged resources through the
IDisposable
interface andusing
statements. - Unsafe code: C# allows for unsafe code blocks where pointer manipulation is possible, but this is discouraged in most scenarios.
While the managed approach of C# simplifies development and reduces certain types of errors, it can introduce performance overhead due to garbage collection and the inability to fine-tune memory usage as precisely as in C++.
Performance Implications
The different memory management models have significant implications for performance:
- C++ generally offers better raw performance and lower memory usage, making it ideal for system-level programming, game engines, and performance-critical applications.
- C# provides good performance for most applications while offering increased productivity and safety. It excels in enterprise applications, web services, and rapid application development scenarios.
Understanding these memory management approaches and their performance implications is crucial when choosing between C++ and C# for a project. The decision often involves balancing the need for absolute performance against development speed and ease of maintenance.
Ecosystem and Platform Support
The ecosystem surrounding a programming language and its platform support are crucial factors that can significantly influence a developer’s or organization’s choice between C++ and C#. These aspects affect not only the development process but also the deployment and maintenance of applications.
C++: Cross-platform and Versatile
C++ boasts a rich and diverse ecosystem that has evolved over decades:
- Cross-platform support: C++ can be compiled for virtually any platform, from embedded systems to supercomputers.
- Standard Template Library (STL): Provides a robust set of container classes, algorithms, and utilities.
- Third-party libraries: A vast array of libraries available for various domains, including Boost, Qt, and OpenCV.
- Build systems: Supports multiple build systems like CMake, Make, and Visual Studio.
- IDEs and tools: Wide range of IDEs including Visual Studio, CLion, and Eclipse CDT.
C++’s ecosystem is characterized by its flexibility and the ability to fine-tune every aspect of the development process. However, this can also lead to fragmentation and complexity in managing dependencies and building processes.
C#: .NET Framework and Beyond
C#’s ecosystem is closely tied to Microsoft’s .NET framework but has expanded significantly in recent years:
- .NET Core/.NET 5+: Cross-platform, open-source implementation of .NET.
- NuGet: Package manager for .NET, simplifying dependency management.
- Visual Studio: Powerful IDE with extensive C# support.
- Xamarin: Framework for building cross-platform mobile applications.
- ASP.NET: Web application framework for building dynamic websites and services.
C#’s ecosystem is more unified and streamlined compared to C++, with strong tooling support from Microsoft. The introduction of .NET Core has significantly expanded C#’s cross-platform capabilities.
Platform Support Comparison
When it comes to platform support, both languages offer extensive options, but with different strengths:
- C++ excels in scenarios requiring low-level system access, embedded systems, and performance-critical applications across all platforms.
- C# shines in Windows development, cross-platform web applications, and increasingly in mobile development through Xamarin.
The choice between C++ and C# often depends on the specific platform requirements of a project, the existing technology stack, and the team’s expertise. C++ offers more flexibility in terms of platform support, while C# provides a more integrated and streamlined development experience, especially within the Microsoft ecosystem.
Use Cases and Industry Adoption
Understanding the typical use cases and industry adoption patterns of C++ and C# is crucial for making informed decisions about which language to use for specific projects or career paths. Both languages have carved out niches in various sectors of the software industry, each excelling in particular domains due to their unique characteristics.
C++: Performance-Critical and System-Level Applications
C++ continues to dominate in areas where performance, low-level control, and system resources are paramount:
- Game Development: Many game engines (e.g., Unreal Engine) and AAA titles are built using C++.
- Operating Systems: Major components of operating systems like Windows, Linux, and macOS are written in C++.
- Embedded Systems: C++ is widely used in IoT devices, automotive software, and other resource-constrained environments.
- High-Frequency Trading: Financial institutions often use C++ for its speed in algorithmic trading systems.
- Graphics and Multimedia: Applications requiring intensive graphics processing often leverage C++.
- Database Engines: Many database systems, including MySQL and MongoDB, use C++ for their core engines.
Industries that heavily adopt C++ include gaming, finance, aerospace, automotive, and scientific computing.
C#: Enterprise Applications and Rapid Development
C# has found its stronghold in enterprise environments and scenarios where development speed and integration with Microsoft technologies are crucial:
- Enterprise Software: Large-scale business applications, especially those integrated with other Microsoft technologies.
- Web Development: ASP.NET is widely used for building web applications and services.
- Windows Desktop Applications: WPF and Windows Forms are popular for creating Windows-specific desktop software.
- Game Development: Unity, a popular game engine, uses C# as its primary scripting language.
- Mobile Development: Xamarin allows C# to be used for cross-platform mobile app development.
- Cloud Services: C# is well-suited for developing cloud-based applications, especially on Azure.
Industries with significant C# adoption include corporate IT, financial services, healthcare, and increasingly, game development (particularly for indie and mobile games).
Comparative Adoption and Trends
While both languages are widely used, their adoption patterns differ:
- C++ maintains a strong presence in industries requiring high performance and low-level control, with a stable or slightly growing user base.
- C# has seen rapid growth, particularly in enterprise environments and with the expansion of .NET Core for cross-platform development.
- Both languages are seeing increased use in emerging fields like machine learning and AR/VR, though often for different components (C++ for performance-critical parts, C# for higher-level application logic).
The choice between C++ and C# often comes down to the specific requirements of the project, the existing technology stack, and the expertise available within the development team. Understanding these use cases and industry trends can guide developers and organizations in making strategic decisions about which language to invest in for current and future projects.
Conclusion: Choosing Between C++ and C#
As we’ve explored the intricacies of C++ and C#, it becomes clear that both languages have their strengths and are suited for different scenarios. The choice between them is not a matter of which is universally “better,” but rather which is more appropriate for specific project requirements, team expertise, and long-term goals.
Key Considerations for Decision-Making
- Performance Requirements: If absolute performance and low-level control are critical, C++ is often the better choice. For applications where slight performance trade-offs are acceptable in favor of rapid development and ease of maintenance, C# may be preferable.
- Development Speed: C# generally offers faster development cycles due to its managed environment and more straightforward syntax. C++ can require more time for development and debugging, especially for complex systems.
- Platform Target: For cross-platform development targeting multiple operating systems and devices, C++ offers more flexibility. However, with .NET Core, C# has significantly improved its cross-platform capabilities, especially for web and cloud applications.
- Team Expertise: The existing skill set of your development team is crucial. Transitioning from C++ to C# is often easier than vice versa due to C#’s simpler memory management model.
- Ecosystem and Libraries: Consider the availability of libraries and frameworks for your specific domain. C++ has a vast ecosystem of specialized libraries, while C# benefits from the extensive .NET framework and its package ecosystem.
- Long-term Maintenance: C# code is generally considered easier to maintain due to its managed nature and more consistent coding practices across projects.
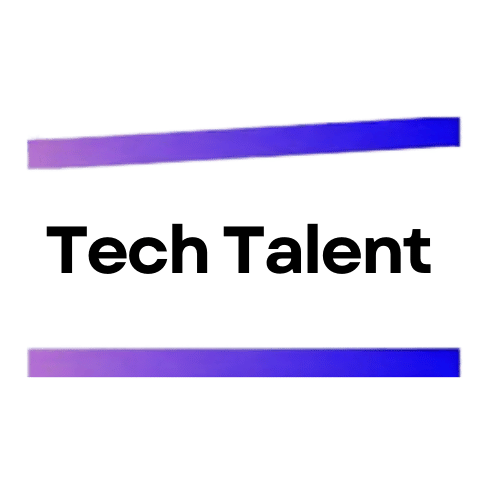
Explore TechTalent: Elevate Your Tech Career
Certify Skills, Connect Globally
TechTalent certifies your technical skills, making them recognized and valuable worldwide.
Boost Your Career Progression
Join our certified talent pool to attract top startups and corporations looking for skilled tech professionals.
Participate in Impactful Hackathons
Engage in hackathons that tackle real-world challenges and enhance your coding expertise.
Access High-Demand Tech Roles
Use TechTalent to connect with lucrative tech positions and unlock new career opportunities.
Visit TechTalent Now!
Explore how TechTalent can certify your skills and advance your tech career!