In the ever-evolving landscape of software development, the ability to write clean and maintainable code is a hallmark of true craftsmanship. It’s not merely about making your code work; it’s about creating a codebase that stands the test of time, welcomes collaboration, and adapts to changing requirements with grace. Let’s delve into why this matters and how it can revolutionize your development process.
Clean code is like a well-organized workspace. Just as a tidy desk can boost productivity, clean code enhances developer efficiency. It’s easier to navigate, understand, and modify. This translates to faster development cycles, reduced debugging time, and smoother onboarding for new team members. Moreover, maintainable code is the bedrock of scalable software. As your project grows, clean code allows for seamless expansion without the fear of introducing bugs or breaking existing functionality.
Consider the long-term implications: a codebase that’s easy to maintain requires less refactoring, fewer rewrites, and ultimately saves time and resources. It’s an investment in the future of your project. Clean code also fosters better collaboration. When your code is clear and well-structured, it becomes a form of communication with other developers, making code reviews more productive and knowledge sharing more effective.
Furthermore, clean and maintainable code is inherently more secure. When code is easy to understand and modify, it’s easier to spot and fix vulnerabilities. This proactive approach to security can save your project from potential disasters down the line. Lastly, writing clean code is a mark of professionalism. It shows respect for your colleagues, your users, and yourself as a developer. It’s a commitment to quality that elevates the entire field of software development.
Following a Consistent Coding Style: The Foundation of Clean Code
Following a consistent coding style is the foundation of writing clean and maintainable code. It’s not just about aesthetics; it’s about creating a unified language within your codebase that speaks volumes about your team’s professionalism and attention to detail. Let’s explore why this practice is crucial and how to implement it effectively.
First and foremost, a consistent coding style dramatically enhances readability. When every piece of code follows the same patterns and conventions, developers can quickly scan and comprehend the logic without getting bogged down by stylistic inconsistencies. This cognitive ease translates to faster development, easier debugging, and more efficient code reviews.
To establish a robust coding style, start by defining clear guidelines. These should cover everything from naming conventions to indentation preferences. For instance, decide whether you’ll use camelCase or snake_case for variable names, and stick to it religiously. Determine your stance on whitespace usage, bracket placement, and comment styles. These may seem like trivial details, but they add up to create a cohesive and professional-looking codebase.
Consistency in architecture is equally vital. Before diving into implementation, outline the architectural principles that will guide your project. This might include decisions about modularity, design patterns, and code organization. By establishing these principles upfront, you ensure that your codebase grows in a structured and predictable manner.
To enforce your chosen style, leverage the power of automated tools. Linters and code formatters are invaluable allies in maintaining consistency. They can catch style violations, suggest improvements, and even automatically format your code to adhere to your guidelines. Popular tools like ESLint for JavaScript or Black for Python can be configured to match your team’s specific style preferences.
Remember, the goal isn’t to debate which style is objectively “best,” but rather to choose a style and apply it consistently. The benefits of consistency far outweigh any perceived advantages of one style over another. By committing to a uniform coding style, you’re laying the groundwork for a codebase that’s not only clean and maintainable but also a joy to work with.
Keeping Functions and Classes Small and Focused: The Art of Modular Design
The principle of keeping functions and classes small and focused is a fundamental tenet of clean code. It’s an approach that embodies the philosophy of “doing one thing, and doing it well.” This practice not only enhances code readability but also significantly improves maintainability and testability. Let’s delve into why this principle is crucial and how to apply it effectively in your coding practices.
At its core, this principle is about modularity and single responsibility. When a function or class has a singular, well-defined purpose, it becomes easier to understand, test, and modify. It’s like having a toolbox where each tool serves a specific purpose, rather than a Swiss Army knife that tries to do everything at once. This clarity of purpose makes your code more intuitive and self-documenting.
To implement this principle, start by scrutinizing your functions and classes. Ask yourself: “Is this doing more than one thing?” If the answer is yes, it’s time to refactor. Break down complex functions into smaller, more focused ones. Each function should have a clear input, output, and a single responsibility. This approach not only makes your code more readable but also more reusable.
Consider the following guidelines when crafting your functions and classes:
- Size matters: Aim for functions that are small enough to fit on a single screen. If you need to scroll to see the entire function, it’s probably doing too much.
- Descriptive naming: Use clear, descriptive names that indicate exactly what the function or class does. This eliminates the need for comments and makes your code self-explanatory.
- Avoid side effects: Functions should ideally not modify state outside their scope. This makes them more predictable and easier to test.
- Limit parameters: If a function requires numerous parameters, it might be a sign that it’s trying to do too much. Consider breaking it down or using object parameters.
Remember, the goal is to create code that’s easy to reason about. When functions and classes are small and focused, they become building blocks that can be easily understood in isolation and then combined to create more complex behavior. This modularity not only makes your code more maintainable but also more flexible and adaptable to change.
Writing Modular and Reusable Code: The Key to Scalability
Writing modular and reusable code is not just a best practice; it’s a paradigm shift in how we approach software development. This principle is about creating code that’s flexible, adaptable, and can stand the test of time. It’s the secret sauce that allows large-scale applications to grow and evolve without becoming unwieldy. Let’s explore why modularity and reusability are crucial and how to incorporate these concepts into your coding practice.
At its essence, modular code is about breaking down complex systems into smaller, manageable pieces. Each module should be self-contained, with a clear interface and minimal dependencies. This approach offers several benefits:
- Improved maintainability: When code is modular, changes can be made to one part of the system without affecting others.
- Enhanced testability: Smaller, focused modules are easier to test in isolation.
- Increased reusability: Well-designed modules can be reused across different parts of an application or even in different projects.
- Better collaboration: Modular code allows different team members to work on separate modules simultaneously.
To write truly modular and reusable code, consider the following strategies:
- Design with interfaces: Use interfaces or abstract classes to define contracts between modules. This allows for loose coupling and makes it easier to swap out implementations.
- Dependency Injection: Instead of hard-coding dependencies, inject them. This makes your code more flexible and easier to test.
- Single Responsibility Principle: Ensure each module has a single, well-defined purpose. This makes modules more reusable and easier to understand.
- Encapsulation: Hide internal details of a module behind a clean, well-defined API. This allows you to change the implementation without affecting code that uses the module.
- Avoid global state: Minimize the use of global variables or singletons. They create hidden dependencies and make code harder to test and reuse.
Remember, writing modular and reusable code is as much about design as it is about implementation. Before you start coding, take the time to plan your module structure. Think about how different parts of your system will interact and how you can design interfaces that are both flexible and easy to use.
Using Comments and Documentation Effectively: Illuminating Your Code
While clean code should strive to be self-explanatory, the judicious use of comments and comprehensive documentation can elevate your codebase from good to exceptional. These tools serve as a bridge between your code and those who interact with it, providing crucial context and insights. Let’s explore how to wield these powerful instruments effectively to enhance the maintainability and usability of your code.
Comments, when used correctly, can provide invaluable insights into the why behind your code, not just the what. They should illuminate complex algorithms, explain non-obvious design decisions, or clarify business logic that might not be immediately apparent from the code alone. However, it’s crucial to strike a balance. Over-commenting can clutter your code and potentially lead to outdated or misleading information if not maintained properly.
Here are some best practices for effective commenting:
- Explain the why, not the how: Your code should be clear enough to explain what it’s doing. Use comments to explain why it’s doing it.
- Keep comments up-to-date: Outdated comments are worse than no comments at all. Make updating comments part of your code review process.
- Use TODO comments judiciously: They can be helpful for marking areas that need future attention, but don’t let them become permanent fixtures.
- Avoid redundant comments: If your code is self-explanatory, additional comments may just add noise.
Documentation, on the other hand, provides a broader context for your code. It should cover everything from high-level architecture to detailed API references. Effective documentation can dramatically reduce onboarding time for new developers and serve as a valuable reference for the entire team.
Consider these strategies for creating effective documentation:
- Use a consistent format: Whether it’s JavaDoc, JSDoc, or another standard, stick to a consistent documentation format throughout your project.
- Include examples: Concrete examples of how to use your code can be incredibly helpful. Consider including code snippets or even full working examples.
- Keep it up-to-date: Like comments, outdated documentation can be misleading. Make updating documentation a part of your development process.
- Use automated tools: Tools like Swagger for API documentation or documentation generators can help maintain consistency and reduce the manual effort required.
- Consider the audience: Different parts of your documentation may be aimed at different audiences (e.g., developers, end-users, system administrators). Tailor your content accordingly.
Remember, the goal of both comments and documentation is to make your code more accessible and understandable. When used effectively, they can significantly enhance the maintainability and usability of your codebase, making it a joy to work with for both you and your fellow developers.
Testing Your Code Rigorously: Ensuring Reliability and Maintainability
Rigorous testing is the bedrock of reliable, maintainable code. It’s not just about catching bugs; it’s about building confidence in your codebase, facilitating refactoring, and providing a safety net for future changes. Let’s delve into why thorough testing is crucial and explore strategies to implement a robust testing regime in your development process.
The benefits of comprehensive testing are manifold:
- Bug Detection: Tests catch errors early in the development cycle when they’re easier and cheaper to fix.
- Code Quality: Writing testable code often leads to better design and more modular, maintainable code.
- Documentation: Tests serve as executable documentation, demonstrating how code is supposed to work.
- Refactoring Confidence: A solid test suite allows you to refactor with confidence, knowing you’ll catch any regressions.
- Collaboration: Tests help new team members understand the codebase and its expected behaviors.
To implement a rigorous testing strategy, consider the following approaches:
- Test-Driven Development (TDD): Write tests before implementing features. This ensures your code is testable from the start and helps clarify requirements.
- Unit Testing: Test individual components in isolation. Aim for high coverage, but focus on critical paths and edge cases.
- Integration Testing: Test how different parts of your system work together. This catches issues that unit tests might miss.
- End-to-End Testing: Test the entire system as a user would interact with it. This ensures all components work together correctly.
- Performance Testing: Regularly test your system’s performance under various conditions to catch performance regressions early.
When implementing your testing strategy, keep these best practices in mind:
- Keep tests fast: Slow tests discourage frequent running. Aim for a test suite that runs in minutes, not hours.
- Make tests independent: Each test should be able to run in isolation. Avoid dependencies between tests.
- Use descriptive test names: A good test name describes the scenario being tested and the expected outcome.
- Don’t test implementation details: Focus on testing behavior, not internal workings. This allows for easier refactoring.
- Embrace test automation: Integrate tests into your CI/CD pipeline to catch issues early and often.
Remember, testing is not a one-time activity but an ongoing process. As your codebase evolves, so should your tests. Regularly review and update your test suite to ensure it remains relevant and effective. By committing to rigorous testing, you’re not just catching bugs; you’re building a foundation for a maintainable, reliable codebase that can stand the test of time.
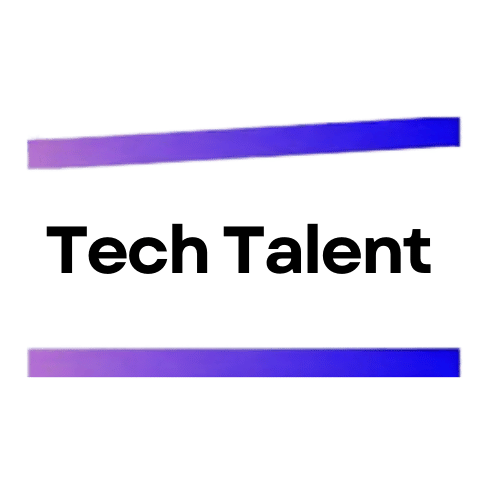
Explore TechTalent: Elevate Your Tech Career
Certify Skills, Connect Globally
TechTalent certifies your technical skills, making them recognized and valuable worldwide.
Boost Your Career Progression
Join our certified talent pool to attract top startups and corporations looking for skilled tech professionals.
Participate in Impactful Hackathons
Engage in hackathons that tackle real-world challenges and enhance your coding expertise.
Access High-Demand Tech Roles
Use TechTalent to connect with lucrative tech positions and unlock new career opportunities.
Visit TechTalent Now!
Explore how TechTalent can certify your skills and advance your tech career!