Secure coding standards form the foundation of cybersecurity practices in software development. These standards encompass a set of guidelines, decisions, and techniques that developers follow across the entire life cycle of software, applications, and websites. Their primary goal is straightforward: to ensure developers create and implement code that protects both the software owner and users by reducing security vulnerabilities.
It’s worth noting that there’s typically more than one way to accomplish any given development task, resulting in varying levels of complexity. Consequently, some solutions inherently offer greater security than others. Secure coding standards serve as a guiding light, steering developers and development teams toward the most secure approach possible, even if it isn’t the fastest route to completion.
While companies and business owners understandably value speedy development and aim to reduce time-to-market, it’s crucial that they remain cognizant of these secure practices for the sake of their livelihood. The news media serves as a stark reminder of the value of these practices, as we frequently witness companies falling victim to data breaches and cyberattacks due to less secure code. Tragically, many of these businesses never fully recover from such incidents.
The OWASP Guidelines: A Blueprint for Secure Coding
In response to the alarming frequency of devastating cyberattacks and the continually evolving methods employed by malicious actors, The Open Web Application Security Project (OWASP) has developed a comprehensive set of guidelines or “best practices” for secure coding in the modern digital landscape. These guidelines serve as an invaluable resource, helping developers maintain the highest level of security throughout the Software Development Life Cycle (SDLC) while preparing for the myriad threats that await once the software is pushed to production.
Among the top practices for secure coding, as outlined by OWASP, are several critical areas that demand attention:
- Password Management: Recognizing passwords as a potential weak point in security, organizations have increasingly adopted multifactor or two-factor authentication. Developers must enforce best practices for password complexity and length, disable password entry after multiple incorrect attempts, and never store passwords in plain text.
- Security by Design: This approach prioritizes security from the outset of development, rather than treating it as an afterthought. It helps reduce future technical debt and mitigates risks proactively.
- Access Control: By defaulting to denial for sensitive data access and restricting privileges to only those who truly need them, companies can significantly reduce the risk of data leaks.
- Data Input Validation: Developers should implement rigorous validation for all input fields, filtering out potentially hazardous characters and ensuring that only accepted data formats are collected.
- System Configuration and Vulnerability Management: Regular updates, patching, and removal of unnecessary components are crucial for maintaining system security.
Implementing Password Management Best Practices
Password management stands as a critical pillar in the edifice of secure coding practices. As passwords often represent the first line of defense against unauthorized access, it’s imperative that developers implement robust strategies to fortify this potential vulnerability. Let’s delve deeper into the best practices for password management in secure coding:
- Enforce Strong Password Policies: Implement stringent password creation rules that require a combination of uppercase and lowercase letters, numbers, and special characters. Additionally, set a minimum password length of at least 12 characters to increase complexity and resistance to brute-force attacks.
- Implement Password Hashing: Never store passwords in plain text. Instead, use strong, slow hashing algorithms like bcrypt, Argon2, or PBKDF2 to securely store password hashes. These algorithms are designed to be computationally intensive, making it significantly more difficult for attackers to crack passwords even if they gain access to the hashed values.
- Salt Passwords: In addition to hashing, always use unique salts for each password. A salt is a random string that is added to the password before hashing, which prevents rainbow table attacks and makes it much harder for attackers to crack multiple passwords simultaneously.
- Implement Account Lockout Mechanisms: After a certain number of failed login attempts (typically 3-5), temporarily lock the account or implement exponential backoff to prevent brute-force attacks. However, be cautious not to create a denial-of-service vulnerability with overly aggressive lockout policies.
- Encourage Use of Password Managers: Promote the use of password managers among users, which can generate and store strong, unique passwords for each account. This reduces the likelihood of password reuse across multiple services.
By meticulously implementing these password management practices, developers can significantly enhance the security posture of their applications and protect users from a wide array of potential threats.
The Security by Design Approach: A Paradigm Shift in Development
The “security by design” approach represents a fundamental shift in the software development paradigm. Rather than treating security as an afterthought or a feature to be bolted on at the end of the development process, this methodology integrates security considerations from the very inception of a project. Let’s explore the key principles and practices that underpin the security by design approach:
- Threat Modeling: Begin each project with a comprehensive threat modeling exercise. This involves identifying potential threats, vulnerabilities, and attack vectors specific to your application. By understanding the risks upfront, you can design appropriate security controls and mitigation strategies.
- Principle of Least Privilege: Design your system architecture and user roles based on the principle of least privilege. This means granting users and system components only the minimum level of access and permissions necessary to perform their functions.
- Defense in Depth: Implement multiple layers of security controls throughout your application. This strategy ensures that if one layer is compromised, additional layers are in place to protect the system.
- Secure Defaults: Configure all system settings, user permissions, and security features to be secure by default. Users should have to explicitly opt-out of security measures rather than opt-in.
- Input Validation and Output Encoding: Implement rigorous input validation on all data entering the system and properly encode all output to prevent injection attacks and cross-site scripting (XSS) vulnerabilities.
- Error Handling: Design error handling mechanisms that provide minimal information to end-users while logging detailed error messages securely for debugging purposes. This prevents information leakage that could be exploited by attackers.
- Continuous Security Testing: Integrate security testing throughout the development lifecycle. This includes static code analysis, dynamic application security testing (DAST), and regular penetration testing.
By embracing the security-by-design approach, development teams can proactively address potential vulnerabilities and security risks, resulting in more robust and resilient software systems. This not only enhances the overall security posture but also reduces the long-term costs associated with addressing security issues post-deployment.
Access Control: Safeguarding Sensitive Data
Access control is a critical component of secure coding practices, serving as a gatekeeper to sensitive data and system resources. Proper implementation of access control mechanisms can significantly reduce the risk of unauthorized access, data breaches, and privilege escalation attacks. Let’s delve into the key principles and best practices for implementing robust access control:
- Principle of Least Privilege (PoLP): This fundamental principle dictates that users, processes, and systems should be granted only the minimum level of access necessary to perform their required functions. Implementing PoLP helps limit the potential damage that can be caused by a compromised account or system component.
- Role-Based Access Control (RBAC): Implement a role-based access control system where permissions are assigned to roles rather than individual users. This approach simplifies access management and reduces the likelihood of privilege creep over time.
- Attribute-Based Access Control (ABAC): For more complex scenarios, consider implementing ABAC, which makes access decisions based on a combination of user attributes, resource attributes, and environmental conditions. This provides finer-grained control over access permissions.
- Multi-Factor Authentication (MFA): Implement MFA for all user accounts, especially those with elevated privileges. This adds an extra layer of security by requiring users to provide multiple forms of identification before granting access.
- Regular Access Reviews: Conduct periodic reviews of user access rights and permissions. This helps identify and revoke unnecessary or outdated access, reducing the attack surface of your application.
- Secure Session Management: Implement secure session handling mechanisms, including the use of secure, randomly generated session tokens, proper session timeout policies, and secure storage of session data.
- Logging and Monitoring: Implement comprehensive logging of all access attempts, both successful and failed. Regularly monitor these logs for suspicious activity and potential security breaches.
Implementing meticulous access control practices enables developers to establish a strong security framework. This framework safeguards sensitive data from unauthorized access and potential breaches. It’s crucial to note that access control isn’t a one-time setup. Instead, it demands continuous management and refinement. This ongoing effort ensures adaptation to evolving security needs and emerging threats.
Conclusion: The Imperative of Secure Coding in Modern Software Development
As we navigate the increasingly complex and treacherous digital landscape, the importance of secure coding practices cannot be overstated. The practices recommended by OWASP are crucial for developers and organizations aiming to create secure software. They include robust password management, security-by-design principles, meticulous access control, and rigorous data input validation. Each of these aspects is essential for strengthening our digital defenses.
However, it’s important to recognize that secure coding is not a static target but a continually evolving discipline. As cyber threats grow more sophisticated, so too must our approaches to security. This necessitates a commitment to ongoing learning, adaptation, and vigilance from all stakeholders in the software development process.
Additionally, prioritizing technical aspects in secure coding is vital. Equally crucial is cultivating a security-focused culture across teams and organizations. This includes training, awareness programs, and fostering an environment that values security throughout the development lifecycle.
In conclusion, adhering to these best practices and maintaining a proactive approach to security can significantly enhance development teams’ capacity to safeguard code and protect user information. This, in turn, helps uphold organizational integrity and safeguard its reputation. In today’s landscape, a single security breach can lead to widespread impacts. Therefore, investing in secure coding practices isn’t just wise but crucial for sustaining long-term success in any software-driven enterprise.
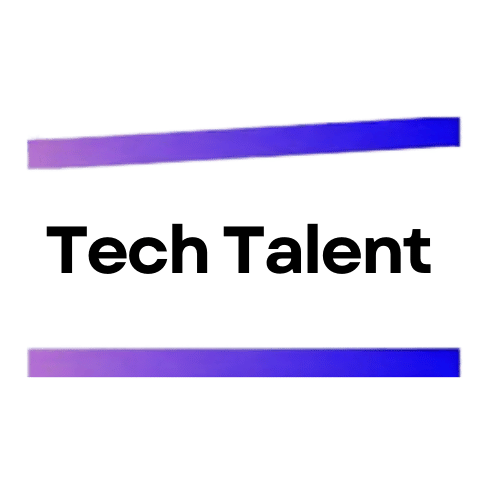
Explore TechTalent: Elevate Your Tech Career
Certify Skills, Connect Globally
TechTalent certifies your technical skills, making them recognized and valuable worldwide.
Boost Your Career Progression
Join our certified talent pool to attract top startups and corporations looking for skilled tech professionals.
Participate in Impactful Hackathons
Engage in hackathons that tackle real-world challenges and enhance your coding expertise.
Access High-Demand Tech Roles
Use TechTalent to connect with lucrative tech positions and unlock new career opportunities.
Visit TechTalent Now!
Explore how TechTalent can certify your skills and advance your tech career!