There are scenarios where injecting custom HTML directly into the page becomes necessary. A prime example is the implementation of a rich-text editor, where we need to display HTML content stored in a database. While this may seem straightforward, Angular’s robust security features can make this task more challenging than anticipated.
When attempting to utilize the DOM’s innerHTML feature in an Angular application to apply HTML to the page, you might find that it doesn’t behave as expected. For instance, inline styles may be stripped away. This article aims to shed light on Angular’s built-in security measures that prevent direct code injection, explain their importance, and demonstrate how to safely inject HTML content into the DOM when necessary.
The Intricacies of innerHTML in Angular
The innerHTML DOM property is a standard feature that allows developers to dynamically inject HTML content into the DOM. It’s important to note that innerHTML is not an Angular-specific directive, but rather a standard DOM functionality. When working on an Angular project and aiming to inject HTML content into the DOM, your initial instinct might be to use the innerHTML property like this:
<div [innerHTML]="htmlContent"></div>
In your component:
export class AppComponent {
htmlContent = "<h1 style="color:red">Hello World</h1>";
}
However, you’ll quickly discover that Angular doesn’t render this as expected. While the h1 tag will be rendered, any inline styles typically applied in a rich text editor will be sanitized. Consequently, the h1 tag will be displayed, but not in the intended red color. This behavior can be perplexing and frustrating for developers unfamiliar with Angular’s security measures.
Unraveling the Mystery: Why Direct innerHTML Use Falls Short in Angular
The reason behind this unexpected behavior lies in Angular’s built-in code injection defenses, also known as XSS (Cross-Site Scripting) defenses. By default, Angular assumes that any text passed to the template is potentially unsafe. As a precautionary measure, it escapes this text according to the context in which it’s being used.
While this might initially seem like an inconvenience, it’s actually a crucial security feature. This escaping mechanism serves as a protective shield, guarding our application against various types of script injection security attacks. It’s a testament to Angular’s commitment to creating secure web applications out of the box.
However, there are legitimate scenarios where bypassing these defenses becomes necessary, such as in our rich-text editor example. Fortunately, Angular provides tools to achieve this safely.
Harnessing DomSanitizer: A Cautious Approach to Bypassing Angular’s Escaping
Angular’s DomSanitizer service offers a suite of methods designed to bypass security checks for trusted values. Here’s a step-by-step guide on how to leverage this service:
- Import DomSanitizer: Begin by importing the DomSanitizer service from the @angular/platform-browser package in the component where you need to bypass escaping.
- Inject DomSanitizer: Add DomSanitizer to your component’s constructor to make it available for use within the component.
- Choose the appropriate sanitization method: DomSanitizer provides several methods, each tailored for different types of content:
- bypassSecurityTrustHtml for HTML
- bypassSecurityTrustStyle for styles
- bypassSecurityTrustScript for script URLs
- bypassSecurityTrustUrl for URL/resource URLs
- bypassSecurityTrustResourceUrl for resource URLs (e.g., iframe sources)
Here’s a comprehensive example demonstrating this approach:
@Component({
selector: "app-unsafe-component",
template: `<div [innerHTML]="trustedHtml"></div>`,
})
export class UnsafeComponent {
trustedHtml: any;
constructor(private sanitizer: DomSanitizer) {
const unsafeHtml = "<h1>Hello World!</h1>";
// Bypassing Angular's HTML sanitizer
this.trustedHtml = this.sanitizer
.bypassSecurityTrustHtml(sanitizedHtml);
}
}
This approach effectively tells Angular to trust the provided content and skip the usual security measures. However, it’s crucial to remember that when bypassing Angular’s escaping, you assume the responsibility of ensuring the content’s safety.
Streamlining HTML Injection: Introducing the SafeHtml Pipe
While the DomSanitizer approach works, it can be somewhat cumbersome to implement repeatedly. To streamline this process, we can create a custom pipe that sanitizes HTML content. This pipe will internally use the DomSanitizer, eliminating the need to use it directly in multiple places within our application.
Here’s how we can create and use such a pipe:
@Pipe({
name: "safeHtml",
standalone: true,
})
export class SafeHtmlPipe {
constructor(private sanitizer: DomSanitizer) {}
transform(html) {
return this.sanitizer.bypassSecurityTrustHtml(html);
}
}
We can then use this pipe in our components as follows:
@Component({
standalone: true,
imports: [SafeHtmlPipe],
template: `
<div [innerHTML]="someHtmlContent | safeHtml">
</div> `,
})
export class TestComponent {}
This approach effectively injects the HTML into the DOM while bypassing Angular’s typical XSS defenses. However, it’s crucial to remember that with great power comes great responsibility. When using this method, you become responsible for ensuring the safety of the injected code.
Conclusion: Balancing Functionality and Security
While Angular’s built-in security measures are invaluable in protecting applications from potential threats, there are scenarios where bypassing these measures becomes necessary. The DomSanitizer service and custom pipes like SafeHtml provide powerful tools for injecting HTML content safely into Angular applications.
However, it’s paramount to exercise caution when using these methods. Always ensure that the content you’re injecting is from a trusted source and has been properly sanitized. Remember, with the power to bypass Angular’s security measures comes the responsibility to maintain the integrity and security of your application.
By understanding these concepts and tools, you can create more dynamic and flexible Angular applications while still maintaining a strong security posture. As you continue to develop and refine your Angular skills, keep these techniques in your toolkit, but always prioritize the security and stability of your applications.
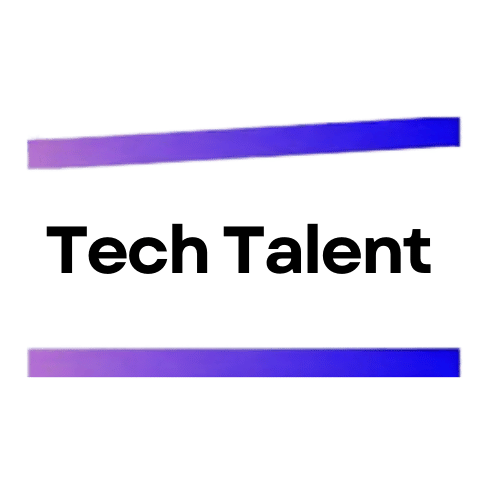
Explore TechTalent: Elevate Your Tech Career
Ready to take your interactive walkthrough skills to the next level?
TechTalent offers opportunities to certify your skills, connect with global tech professionals, and explore interactive design and development.
Join today and be part of shaping the future of interactive walkthroughs!