Before delving into React Basics, starting your journey with React necessitates a strong understanding of fundamental web development concepts. Mastery of HTML, CSS, and JavaScript is essential as these lay the groundwork for advancing your React expertise.
HTML (Hypertext Markup Language) provides the structure for your web pages. It’s the skeleton that holds everything together. Familiarize yourself with semantic HTML5 tags like <header>
, <nav>
, and <article>
to create meaningful, accessible content structures. This knowledge will prove invaluable when you start crafting React components.
CSS (Cascading Style Sheets) breathes life into your HTML structure. Master the art of styling with CSS selectors, flexbox, and grid layouts. Understanding CSS modules and styled components will be particularly beneficial when you start styling React components. Don’t shy away from exploring CSS preprocessors like Sass or Less, as they can significantly enhance your styling workflow.
JavaScript is the linchpin of React development. Ensure you’re comfortable with ES6+ features such as arrow functions, destructuring, and the spread operator. These modern JavaScript concepts are ubiquitous in React codebases. Delve into asynchronous programming with Promises and async/await syntax, as these are crucial for handling data fetching and other side effects in React applications.
Once you’ve fortified your web development foundation, it’s time to immerse yourself in the React ecosystem. Start by perusing the official React documentation, which offers a comprehensive introduction to React’s core concepts. As you progress, consider enrolling in a structured course that aligns with your experience level and learning style. Remember, the key to mastering React is consistent practice and application of concepts.
Thinking in React: A Paradigm Shift in UI Development
The “Thinking in React” approach is a fundamental paradigm shift in how developers conceptualize and construct user interfaces. This methodology encourages breaking down complex UIs into smaller, reusable components, fostering a modular and maintainable codebase. By adopting this mindset, you’ll find yourself creating more scalable and efficient React applications.
At the heart of “Thinking in React” is the component lifecycle. Each React component goes through a series of phases from creation to unmounting. Understanding these lifecycle methods is crucial for managing side effects, optimizing performance, and ensuring proper resource cleanup. For instance, the componentDidMount()
method is ideal for initiating API calls or setting up subscriptions, while componentWillUnmount()
is perfect for cleaning up those subscriptions to prevent memory leaks.
The declarative nature of React is another cornerstone of this approach. Instead of imperatively manipulating the DOM, you describe what you want the UI to look like at any given point. React then efficiently updates and renders the right components when your data changes. This declarative paradigm leads to more predictable and easier-to-debug code.
As you delve deeper into “Thinking in React,” you’ll encounter the concept of unidirectional data flow. This principle stipulates that data in your application should flow in a single direction, typically from parent components to child components via props. This approach simplifies state management and makes it easier to track where and how data changes in your application.
To truly master “Thinking in React,” practice decomposing complex UIs into component hierarchies. Start with a mockup of your desired interface and identify the logical components within it. Consider which components should be stateful and which should be purely presentational. This exercise will hone your ability to design efficient and reusable component structures.
React Components: Building Blocks of Modern UIs
React components are the fundamental building blocks of any React application. They encapsulate pieces of your user interface, making your code more modular, reusable, and maintainable. There are two primary types of components in React: class components and function components.
Class components, defined using ES6 classes, were the traditional way of creating components in React. They offer a rich set of lifecycle methods and the ability to maintain internal state. A typical class component might look like this:
class Welcome extends React.Component {
render() {
return Hello, {this.props.name}
;
}
}
Function components, on the other hand, are simpler and more concise. With the introduction of Hooks in React 16.8, function components can now handle state and side effects, making them the preferred choice for many developers. Here’s an example of a function component:
function Welcome(props) {
return Hello, {props.name}
;
}
Props (short for properties) are a crucial concept in React. They allow you to pass data from parent components to child components, enabling dynamic and reusable components. Props are read-only and help maintain the unidirectional data flow in React applications.
The state represents the internal data of a component that can change over time. In class components, state is managed using the setState()
method. In function components, the useState
hook provides state management capabilities. Understanding when to use props vs. state is key to designing efficient React components.
The Virtual DOM: React’s Performance Powerhouse
The Virtual DOM (Document Object Model) is one of React’s most innovative features, serving as the cornerstone of its impressive performance. This abstraction of the actual DOM allows React to optimize rendering and updates, resulting in faster and more efficient user interfaces.
At its core, the Virtual DOM is a lightweight JavaScript representation of the actual DOM. When changes occur in a React application, React first updates this virtual representation. It then uses a diffing algorithm to compare the updated Virtual DOM with the previous version, identifying the minimal set of changes needed to update the actual DOM.
This process, known as reconciliation, is what gives React its performance edge. Instead of re-rendering the entire DOM tree for every change, React only updates the parts that have actually changed. This approach significantly reduces the computational overhead associated with DOM manipulation, leading to smoother and more responsive user interfaces.
To leverage the power of the Virtual DOM effectively, it’s important to understand React’s rendering process. When a component’s state or props change, React schedules a re-render of that component and its children. However, this doesn’t mean that the actual DOM is immediately updated. React batches multiple updates together and performs them asynchronously for optimal performance.
As a developer, you can optimize your React applications by minimizing unnecessary re-renders. Techniques such as using the shouldComponentUpdate
lifecycle method in class components or the React.memo
higher-order component for function components can help prevent superfluous rendering cycles, further enhancing your application’s performance.
Styling in React: Crafting Visually Appealing UIs
Styling in React offers a plethora of options, each with its own strengths and use cases. From traditional CSS to more React-specific solutions, understanding these approaches is crucial for creating visually appealing and maintainable user interfaces.
CSS Modules is a popular styling approach in the React ecosystem. It allows you to write CSS that’s scoped to a specific component, preventing style conflicts across your application. Here’s how you might use CSS Modules in a React component:
import styles from './Button.module.css';
function Button() {
return ;
}
Styled-components is another powerful styling solution that leverages tagged template literals to style your components. This approach allows you to write actual CSS within your JavaScript files, promoting a component-centric styling approach:
import styled from 'styled-components';
const Button = styled.button`
background-color: blue;
color: white;
padding: 10px 20px;
`;
function MyComponent() {
return ;
}
For those who prefer a utility-first approach, Tailwind CSS has gained significant popularity in the React community. It provides low-level utility classes that you can compose to build custom designs without leaving your HTML (or JSX in React’s case):
function Button() {
return (
);
}
Regardless of the styling approach you choose, it’s important to maintain consistency throughout your application. Consider creating a design system or component library to ensure a cohesive look and feel across your React projects.
Advancing Your React Journey: Next Steps and Best Practices
As you progress in your React journey, there are several advanced concepts and best practices to explore. These will not only enhance your skills but also make you a more effective and efficient React developer.
State management becomes increasingly important as your applications grow in complexity. While React’s built-in state management is sufficient for many cases, you might find yourself needing more robust solutions for larger applications. Redux is a popular state management library that works well with React, providing a predictable state container for JavaScript apps. Alternatively, you might explore the Context API, which is built into React and can be a simpler solution for many state management needs.
Testing is a crucial aspect of any serious development process. Jest is a popular testing framework that works seamlessly with React applications. Combined with React Testing Library, it allows you to write comprehensive unit and integration tests for your components. Here’s a simple example of a Jest test for a React component:
import { render, screen } from '@testing-library/react';
import MyComponent from './MyComponent';
test('renders learn react link', () => {
render();
const linkElement = screen.getByText(/learn react/i);
expect(linkElement).toBeInTheDocument();
});
As you become more comfortable with React, consider exploring some of the popular frameworks built on top of it. Next.js, for instance, is a powerful framework for server-side rendered React applications. It provides features like automatic code splitting, optimized prefetching, and static site generation out of the box.
Lastly, don’t forget about performance optimization. React provides tools like the Profiler API to help you identify performance bottlenecks in your application. Learn to use React.memo for preventing unnecessary re-renders, and explore techniques like code splitting and lazy loading to improve your application’s load time.
Remember, mastering React is a journey, not a destination. Stay curious, keep practicing, and don’t hesitate to dive into the vibrant React community for support and inspiration. Happy coding!
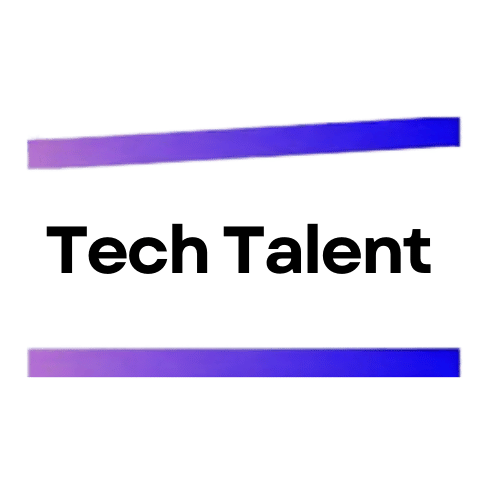
Explore TechTalent: Elevate Your Tech Career
Ready to take your interactive walkthrough skills to the next level?
TechTalent offers opportunities to certify your skills, connect with global tech professionals, and explore interactive design and development.
Join today and be part of shaping the future of interactive walkthroughs!