Angular performance tuning is paramount in the ever-evolving landscape of web development. This comprehensive guide dives into the intricacies of bundle size optimization, empowering you to create lightning-fast Angular applications that leave users in awe.
Angular, renowned for its robustness and versatility, can sometimes fall prey to performance bottlenecks, particularly when it comes to bundle sizes. Fear not, for we’re about to embark on a journey that will transform your Angular application from a sluggish behemoth into a lean, mean, performance machine.
Our odyssey begins with the art of performance profiling, where we’ll uncover the hidden culprits lurking within your application’s bundle. Armed with this knowledge, we’ll then delve into the cutting-edge techniques of bundle size optimization, including standalone lazy-loading and partial template loading. These powerful tools will revolutionize the way you approach Angular development, ensuring your applications are not just functional, but blazingly fast.
So, fasten your seatbelts and prepare to elevate your Angular expertise to new heights. Let’s dive into the world of Angular Performance Tuning and unlock the secrets to creating applications that are not just good, but truly exceptional.
Mastering the Art of Performance Profiling
Before we can optimize our Angular application, we need to understand where the performance bottlenecks lie. This is where the art of performance profiling comes into play. It’s not about guesswork or intuition; it’s about cold, hard data that illuminates the path to optimization.
Enter the source-map-explorer package, a powerful tool that generates a visual report of your bundle’s disk space usage. This invaluable resource provides a detailed breakdown of the libraries and Angular components that are consuming the most space in your JavaScript bundle.
To harness the power of source-map-explorer, follow these steps:
- Install the package globally using npm:
npm install -g source-map-explorer
- Add the following npm script to your project’s package.json:
"bundle-report": "ng build --configuration production --source-map && source-map-explorer dist/browser/*.js"
- Run the script to generate your bundle report:
npm run bundle-report
This script performs two crucial tasks: first, it creates a production build of your application with source maps enabled. Then, it utilizes source-map-explorer to generate a comprehensive report based on these source maps.
The resulting report is a treasure trove of information, revealing which components and libraries are the heaviest contributors to your bundle size. Armed with this knowledge, you can make informed decisions about which parts of your application are prime candidates for optimization techniques like lazy loading.
Remember, the key to effective optimization is to focus on the low-hanging fruit first. Look for rarely used but heavyweight dependencies, such as PDF generation libraries or complex charting tools. These are often ideal candidates for code splitting, as they can be loaded on-demand rather than burdening your initial bundle.
Embracing the Power of Lazy Loading
Now that we’ve identified the performance bottlenecks in our Angular application, it’s time to unleash the full potential of lazy loading. The goal is to create a fully lazy-loaded application, where each screen is loaded in a separate bundle, dramatically reducing the initial load time and improving overall performance.
The latest versions of Angular have made this process incredibly straightforward, thanks to the introduction of standalone components. These self-contained units of functionality can be easily lazy-loaded, simplifying our optimization efforts.
Let’s examine how we can transform a regular route into a lazy-loaded one using standalone components:
Before (eager loading):
export const routes: Routes = [
{
path: 'courses/:courseUrl',
component: WatchCourseComponent
}
]
After (lazy loading):
export const routes: Routes = [
{
path: 'courses/:courseUrl',
loadComponent: () =>
import('./watch-course/watch-course.component')
.then(mod => mod.WatchCourseComponent)
}
]
This simple change moves the WatchCourseComponent and all its dependencies into a separate bundle, which is loaded only when the user navigates to that specific route. The beauty of this approach is its simplicity and effectiveness in reducing the main bundle size.
To fully optimize your application, apply this technique to all your routes. The result will be a lean main bundle containing primarily the Angular framework itself, with individual feature bundles loaded on-demand as the user navigates through your application.
This approach not only improves initial load times but also enhances the overall user experience by ensuring that users only download the code they need when they need it.
Unleashing the Power of Partial Template Loading
While lazy loading at the route level is a powerful optimization technique, we can push the boundaries of performance even further with partial template loading. This advanced technique allows us to lazy load specific parts of a component’s template, providing granular control over what code is loaded and when.
Angular’s innovative @defer directive is the key to implementing partial template loading. This feature allows us to conditionally load parts of our template based on specific triggers or conditions, further optimizing our application’s performance.
Consider a scenario where we have a course player component that supports various lesson types, such as video, audio, and digital downloads. Instead of loading all the code for these different lesson types upfront, we can use @defer to load only what’s necessary based on the current lesson type:
@defer(when lesson.type == 'audio') {
@if(lesson.type == 'audio') {
}
}
In this example, the audio player component and its associated code will only be loaded when the lesson type is ‘audio’. This prevents unnecessary code from being downloaded and parsed when it’s not needed, further reducing the initial load time and improving the application’s responsiveness.
The @defer directive offers a range of options for controlling when and how content is loaded, including:
- on idle: Load the content when the browser is idle
- on viewport: Load when the element enters the viewport
- on interaction: Load when the user interacts with a specific element
- when: Load based on a custom condition
By strategically applying @defer throughout your application, you can create a highly optimized user experience where code is loaded precisely when it’s needed, and not a moment sooner.
Implementing a Systematic Optimization Approach
Now that we’ve explored the powerful tools at our disposal for Angular performance tuning, it’s time to implement a systematic approach to optimization. This iterative process will help you continuously improve your application’s performance over time.
Here’s a step-by-step guide to implementing a robust optimization strategy:
- Generate a Performance Profile: Start by creating a bundle report using the source-map-explorer tool. This will give you a clear picture of your application’s current state and help identify the most significant performance bottlenecks.
- Implement Route-Level Lazy Loading: Leverage standalone components and the loadComponent syntax to make every screen in your application lazy-loaded. This will significantly reduce your main bundle size and improve initial load times.
- Apply Partial Template Loading: Use the @defer directive to lazy load specific parts of your components’ templates. Focus on large or rarely used features that can be loaded on-demand.
- Re-profile and Iterate: After implementing these optimizations, generate a new bundle report. Compare it to the original to measure your progress and identify any remaining bottlenecks.
- Optimize Third-Party Dependencies: Look for opportunities to replace heavy libraries with lighter alternatives, or consider implementing your own streamlined solutions for specific features.
- Implement Code Splitting: For larger applications, consider splitting your code into feature modules that can be loaded independently.
- Optimize Assets: Don’t forget about non-JavaScript assets. Compress images, use appropriate formats (e.g., WebP), and leverage lazy loading for images and other media.
- Monitor Real-World Performance: Implement performance monitoring in your production environment to catch any issues that may not be apparent during development.
Remember, performance optimization is an ongoing process. As your application evolves and grows, new performance challenges may arise. By consistently reviewing these steps and keeping abreast of the newest Angular features and best practices, you can maintain your application’s speed and responsiveness. This will enhance user satisfaction with every interaction.
Conclusion: Elevating Your Angular Application to New Heights
Optimizing bundle size is more than a technical exercise—it’s an art form that can enhance your application’s user experience. By implementing strategies like performance profiling, lazy loading, and partial template loading, you’ll create Angular applications that are both functional and exceptionally fast.
Remember, the journey to optimal performance is ongoing. As your application evolves, so too should your optimization strategies. Stay curious, keep experimenting, and always be on the lookout for new ways to squeeze every ounce of performance out of your Angular applications.
By mastering these techniques, you’re improving load times and crafting delightful user experiences. This will set your applications apart in a competitive digital landscape. Optimize with confidence and watch your Angular applications soar to new heights of performance and user satisfaction.
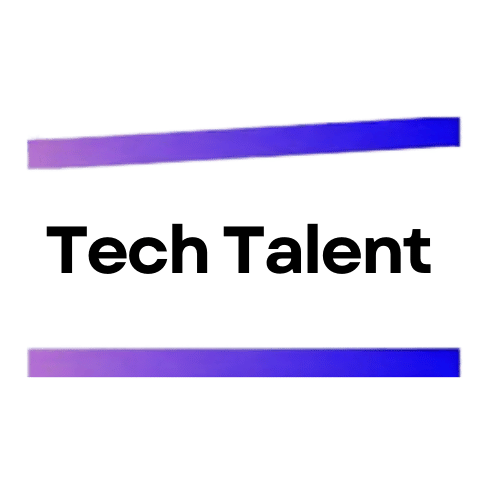
Explore TechTalent: Elevate Your Tech Career
Ready to take your interactive walkthrough skills to the next level?
TechTalent offers opportunities to certify your skills, connect with global tech professionals, and explore interactive design and development.
Join today and be part of shaping the future of interactive walkthroughs!